문제조건
1. 현재 시간을 출력
2. 추가할 초를 입력받는다.
3. 2번 단계에 소요된 시간이 2번에서 추가하겠다고 입력한 초보다 길면, 바로 Time is over 출력
4. 2번 단계에 소요된 시간이 2번에서 추가하겠다고 입력한 초보다 짧으면(=숫자를 빨리입력후 엔터눌렀으면) 입력된 숫자만큼 초가 경과한 후에 Time is over 출력
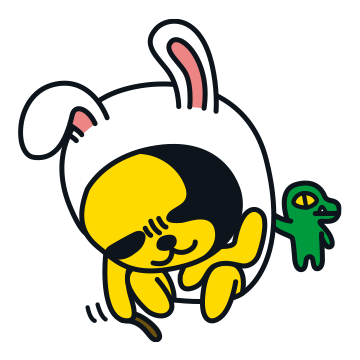
※그냥 시간을 멈추면 되는게 아니라서 되게 까다로운 타이머다..
알고리즘
초를 어떻게 계산해야하나 엄청 고민했다. 원하는 형태로 출력되지않아서 이틀동안 고민해서 풀었다. 덕분에 currentTimeMillis() 함수를 열심히 다뤘다.
public class iam_im2 {
public static void main(String[] args) {
calTime();
}
public static void calTime() {
// 1. 처리전 시간 획득
long start = System.currentTimeMillis();
System.out.print("Now : ");
System.out.println(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS").format(start));
// 2. 처리할 작업
Scanner sc = new Scanner(System.in);
System.out.println("Enter the seconds you want to add : ");
long inputNum = sc.nextInt();
System.out.println("");
// 3. 처리했어야할 시간 획득
long upToTime = start + (inputNum * 1000);
System.out.println("Must be entered by this time: " + new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS").format(upToTime));
// 4. 처리후 시간 획득
long end = System.currentTimeMillis();
System.out.print("Now : ");
System.out.println(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS").format(end));
// 5. 시간차이 출력_숫자입력까지 실제로 걸린시간
System.out.println("Time taken to enter a number : " + (end - start) / 1000.0 + " seconds\n");
// 6. 시간차이 계산
if ((end - start) / 1000.0 > (upToTime - start) / 1000.0) {
System.out.println("Time is over");
System.out.println("current time : " + new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS").format(end));
} else if ((end - start) / 1000.0 <= (upToTime - start) / 1000.0) { // 입력한시간보다 더 빠르게 입력했으면=아직 마감시간 전이라면
try {
Thread.sleep(inputNum * 1000);
} catch (InterruptedException e) {
e.printStackTrace();
} // 입력시간만큼 지난후에 문구 출력
System.out.println("Time is over");
System.out.println("current time : " + new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS").format(upToTime));
System.out.println("");
}
}
}
/*
Now : 2022-04-10 02:29:39.169
Enter the seconds you want to add :
10
Must be entered by this time: 2022-04-10 02:29:49.169
Now : 2022-04-10 02:29:41.415
Time taken to enter a number : 2.246 seconds
Time is over
current time : 2022-04-10 02:29:49.169
*/
<풀이 과정>
1. 현재시간을 구한다.
2. 추가할 시간(inputNum)을 입력받는다.
3. 입력이 완료된 시점의 시간을 구한다.
4. 입력이 완료된 시점의 시간이 (현재시간 + inputNum) 보다 늦으면(즉, 사용자가 시간을 늦게 입력했으면) 바로 Time is over 출력
5. 입력이 완료된 시점의 시간이 (현재시간 + inputNum) 보다 빠르면(즉, 사용자가 해당 시간이 되기전에 입력했으면), inputNum만큼 기다려서 목표시간에 도달하면 그때 Time is over 출력
<계산에 사용할 함수 선택>
Date, Calendar 클래스보다 currentTimeMillis() 가 더 정밀하다. 초 단위를 구해야하고 시작시간, 종료시간 차를 확보해야하므로 currentTimeMillis()를 활용했다.
처음에는 Date와 Calendar클래스를 이용해서 더하기 빼기, Thread를 분리해가며 별짓 다해봤다. 그러다가 Stack Overflow에서 어느 답변에 Calendar 클래스는 멀티쓰레드용으로 개발된 게 아니라서 멀티쓰레드에 사용하면 Calendar인스턴스가 훼손된다는 무시무시한 답변을 봤다. 덕분에 currentTimeMillis()를 활용해서 문제를 풀 수 있었다.
'언어 > JAVA' 카테고리의 다른 글
Eclipse 이클립스 폰트 변경 (0) | 2022.04.14 |
---|---|
가위바위보(Rock-Paper-Scissors Game) (0) | 2022.04.07 |
currentTimeMillis() 함수: 소요시간 계산 (0) | 2022.04.07 |
Arrays.sort 로 배열 정렬하기 (0) | 2022.04.06 |
ArrayList remove 특정 값 삭제 (0) | 2022.04.06 |